3 Tier and MVC dot net Architecture training
Posted By:ExcelPTP
August 21,2013
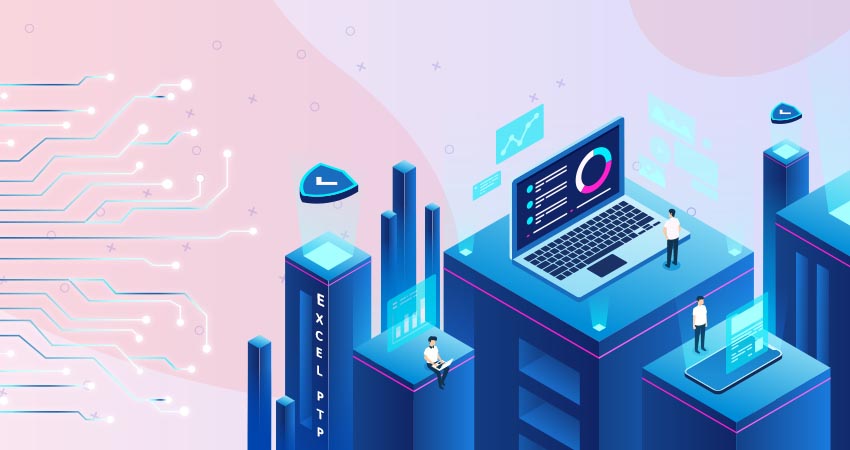
What is Three Tier or N Tier Architecture ?
In Programming Style, All Software Engineer called Three Tie architecture as multi-tier architecture (often referred to as n-tier architecture) is a client–server architecture in which presentation, application processing, and data management functions are logically separated. For example, an application that uses middleware to service data requests between a user and a database employs multi-tier architecture. The most widespread use of multi-tier architecture is the three-tier architecture.
N-tier application architecture provides a model by which developers can create flexible and reusable applications. By segregating an application into tiers, developers acquire the option of modifying or adding a specific layer, instead of reworking the entire application. Three-tier architecture is typically composed of a presentation tier, a business or data access tier, and a data tier.
While the concepts of layer and tier are often used interchangeably, one fairly common point of view is that there is indeed a difference. This view holds that a layer is a logical structuring mechanism for the elements that make up the software solution, while a tier is a physical structuring mechanism for the system infrastructure.
User Interface tier
This is a chunk of code from the user interface. I am only including the functions that are used to call the middle tier or the business logic layer.
I am keeping a reference to business logic layer as BOCustomer
.
//This function get the details from the user via GUI /ExcelPTP*
//tier and calls the Add method of business logic layer.
private void cmdAdd_Click(object sender, System.EventArgs e)
{
try
{
cus = new BOCustomer();
cus.cusID=txtID.Text.ToString();
cus.LName = txtLName.Text.ToString();
cus.FName = txtFName.Text.ToString();
cus.Tel= txtTel.Text.ToString();
cus.Address = txtAddress.Text.ToString();
cus.Add();
}
catch(Exception err)
{
MessageBox.Show(err.Message.ToString());
}
}
//This function gets the ID from the user and finds the
//customer details and return the details in the form of
//a dataset via busniss object layer. Then it loops through
//the content of the dataset and fills the controls.
private void cmdFind_Click(object sender, System.EventArgs e)
{
try
{
String cusID = txtID.Text.ToString();
BOCustomer thisCus = new BOCustomer();
DataSet ds = thisCus.Find(cusID);
DataRow row;
row = ds.Tables[0].Rows[0];
//via looping
foreach(DataRow rows in ds.Tables[0].Rows )
{
txtFName.Text = rows[“CUS_F_NAME”].ToString();
txtLName.Text = rows[“CUS_L_NAME”].ToString();
txtAddress.Text = rows[“CUS_ADDRESS”].ToString();
txtTel.Text = rows[“CUS_TEL”].ToString();
}
}
catch (Exception err)
{
MessageBox.Show(err.Message.ToString());
}
}
//this function used to update the customer details.
private void cmdUpdate_Click(object sender,
System.EventArgs e)
{
try
{
cus = new BOCustomer();
cus.cusID=txtID.Text.ToString();
cus.LName = txtLName.Text.ToString();
cus.FName = txtFName.Text.ToString();
cus.Tel= txtTel.Text.ToString();
cus.Address = txtAddress.Text.ToString();
cus.Update();
}
catch(Exception err)
{
MessageBox.Show(err.Message.ToString());
}
}
Business Logic layer
Here, I am including all the code for this tier. Basically it has properties that are needed to define the customer object. But as I mentioned it is just a dummy customer and many other properties can be added if required. It also has all the methods including Add, update, find that are required to maintain customer details.
This is the middle tier and acts between the GUI and the Data access layer. It keeps a reference to the data access tier as cusData = new DACustomer()
. It also has a reference to System.Data
namespace as sometimes it returns details in the form of DataSet
to the GUI tier.
using System;
using System.Data;
namespace _3tierarchitecture
{
///
/// Summary description for BOCustomer.
///
public class BOCustomer
{
//Customer properties
private String fName;
private String lName;
private String cusId;
private String address;
private String tel;
private DACustomer cusData;
public BOCustomer()
{
//An instance of the Data access layer!
cusData = new DACustomer();
}
///
/// Property FirstName (String)
///
public String FName
{
get
{
return this.fName;
}
set
{
try
{
this.fName = value;
if (this.fName == “”)
{
throw new Exception(
“Please provide first name …”);
}
}
catch(Exception e)
{
throw new Exception(e.Message.ToString());
}
}
}
///
/// Property LastName (String)
///
public String LName
{
get
{
return this.lName;
}
set
{
//could be more checkings here eg revmove ‘ chars
//change to proper case
//blah blah
this.lName = value;
if (this.LName == “”)
{
throw new Exception(“Please provide name …”);
}
}
}
///
/// Property Customer ID (String)
///
public String cusID
{
get
{
return this.cusId;
}
set
{
this.cusId = value;
if (this.cusID == “”)
{
throw new Exception(“Please provide ID …”);
}
}
}
///
/// Property Address (String)
///
public String Address
{
get
{
return this.address;
}
set
{
this.address = value;
if (this.Address == “”)
{
throw new Exception(“Please provide address …”);
}
}
}
///
/// Property Telephone (String)
///
public String Tel
{
get
{
return this.tel;
}
set
{
this.tel = value;
if (this.Tel == “”)
{
throw new Exception(“Please provide Tel …”);
}
}
}
///
/// Function Add new customer. Calls
/// the function in Data layer.
///
public void Add()
{
cusData.Add(this);
}
///
/// Function Update customer details.
/// Calls the function in Data layer.
///
public void Update()
{
cusData.Update(this);
}
///
/// Function Find customer. Calls the
/// function in Data layer.
/// It returns the details of the customer using
/// customer ID via a Dataset to GUI tier.
///
public DataSet Find(String str)
{
if (str == “”)
throw new Exception(“Please provide ID to search”);
DataSet data = null;
data = cusData.Find(str);
return data;
}
}
}
Data Access Layer
The data tier has details to manipulate an MS Access database. However, all these details are transparent and do not affect the business logic layer. This module has a reference to the business logic layer as BOCustomer cus
. To make the usage easier and be supported by any other database I have created some constants including the database name and field name that need to be changed as per the customer table details. This is an usable module for any database after making these changes.
using System; using System.Data.OleDb; using System.Data; namespace _3tierarchitecture { /// /// Summary description for DACustomer. /// public class DACustomer { private OleDbConnection cnn; //change connection string as per the //folder you unzip the files private const string CnnStr = “Provider=Microsoft.Jet.OLEDB.4.0;Data ” + “Source= D:\\Rahman_Backup\\Programming\\” + “Csharp\\3tierarchitecture\\customer.mdb;”; //local variables private String strTable=””; private String strFields=””; private String strValues=””; private String insertStr=””; //this needs to be changed based on customer //table fields’ Name of the database! private const String thisTable = “tblCustomer”; private const String cus_ID = “CUS_ID”; private const String cus_LName = “CUS_L_NAME”; private const String cus_FName = “CUS_F_NAME”; private const String cus_Tel = “CUS_TEL”; private const String cus_Address = “CUS_ADDRESS”; public DACustomer() { } public DACustomer(BOCustomer cus) { // A reference of the business object class } //standard dataset function that adds a new customer public void Add(BOCustomer cus) { String str = BuildAddString(cus); OpenCnn(); //Open command option – cnn parameter is imporant OleDbCommand cmd = new OleDbCommand(str,cnn); //execute connection cmd.ExecuteNonQuery(); // close connection CloseCnn(); } //standard dataset function that updates //details of a customer based on ID public void Update(BOCustomer cus) { OpenCnn(); String selectStr = “UPDATE ” + thisTable + ” set ” + cus_LName + ” = ‘” + cus.LName + “‘” + “, ” + cus_FName + ” = ‘” + cus.FName + “‘” + “, ” + cus_Address + ” = ‘” + cus.Address + “‘” + “, ” + cus_Tel + ” = ‘” + cus.Tel + “‘” + ” where cus_ID = ‘” + cus.cusID + “‘”; OleDbCommand cmd = new OleDbCommand(selectStr,cnn); cmd.ExecuteNonQuery(); CloseCnn(); } //standard dataset function that finds and //return the detail of a customer in a dataset public DataSet Find(String argStr) { DataSet ds=null; try { OpenCnn(); String selectStr = “select * from ” + thisTable + ” where cus_ID = ‘” + argStr + “‘”; OleDbDataAdapter da = new OleDbDataAdapter(selectStr,cnn); ds = new DataSet(); da.Fill(ds,thisTable); CloseCnn(); } catch(Exception e) { String Str = e.Message; } return ds; } private void OpenCnn() { // initialise connection String cnnStr = CnnStr; cnn = new OleDbConnection(cnnStr); // open connection cnn.Open(); } private void CloseCnn() { // 5- step five cnn.Close(); } // just a supporting function that builds // and return the insert string for dataset. private String BuildAddString(BOCustomer cus) { // these are the constants as // set in the top of this module. strTable=”Insert into ” + thisTable; strFields=” (” + cus_ID + “,” + cus_LName + “,” + cus_FName + “,” + cus_Address + “,” + cus_Tel + “)”; //these are the attributes of the //customer business object. strValues= ” Values ( ‘” + cus.cusID + “‘ , ‘” + cus.LName + “‘ , ‘” + cus.FName + “‘ , ‘” + cus.Address + “‘ , ‘” + cus.Tel + “‘ )”; insertStr = strTable + strFields + strValues; return insertStr; } } }
Eligibility & Course Duration
You can take this training only with having basic computer knowledge or we can clear that up for you. The total duration for the course is 4 to 6 months (5 days a week 4 hours / full day only practical things)
- MCA / BCA / MBA IT Candidates
- BE /B tech / IT / EC candidates
- Msc IT / Bsc IT Candidates
- Diploma in Computer Engg. Candidates can apply
- Others graduate too for non Technical IT courses.
Live Project Training
The course doesn’t end here, every student once completed the course successfully has to work on an international live project under a technical in some of the reputed IT companies. This strongly helps students of MCA, BE, BCA, MSc IT and BSc IT to have their final semester project completed as well gives a practical experience working under with an IT company.
Small Task, Demo & Live Project-:
It is very compulsory to work on an international live project under a technician in some of the reputed IT companies. This helps students of MCA, BE, BCA, MSc IT and BSc IT to have their final semester project completed as well gives a practical experience working under with an IT company.
For more information you can contact us at our email Ids as: info@ExcelPTP.com or visit our Office at office Address.